Canvas

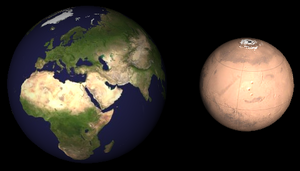
The Canvas component lets you render 2D and 3D shapes, as well as bitmap images, dynamically.
It consists of a rectangular surface where you can draw graphics, either shapes or images (from files), using JavaScript. All the drawing is performed on the fly, letting you change the content of the canvas as a reaction to user input.
You can use the canvas to draw graphs, create animations, compose photos, or even develop games; all without relying on heavy libraries or client-side plugins like Flash.
Usage
To use a Canvas, you only need to define and event handler for its OnPaint JavaScript event. Use that event to retrieve the canvas context (event parameter) and use it to draw on the canvas.
For example:
function ComponentNameJSPaint($sender, $params)
{
?>
// Get context and canvas component.
context = event;
canvas = $('#ComponentName');
// Draw a black circle on the center.
context.beginPath();
context.arc(canvas.width/2, canvas.height/2, 100, 0, 2 * Math.PI, false);
context.fillStyle = "#000";
context.fill();
context.closePath();
<?php
}
The context is available outside the event, by the name of ComponentName_ctx, where the prefix ComponentName should be replaced by the actual Name of your canvas component. For example: Canvas1_ctx.
Clear
To clear the canvas, you can use the clearRect()
method of the context:
context = ComponentName_ctx;
canvas = $('#ComponentName');
context.clearRect(0, 0, canvas.width, canvas.height);
Client-side Features
DOM Elements
The component generates the following client-side DOM elements:
- Wrapper (HTMLDivElement). Full web browser support. Access it with
$("#ComponentName_outer").get()[0]
.- Main element (HTMLCanvasElement). Wide browser support. Access it with:
$("#ComponentName").get()[0]
.
- Main element (HTMLCanvasElement). Wide browser support. Access it with:
Client-side Events
- Documented in the RPCL Reference.
Client-side Functions
Note: In order to call these functions, prefix them with
ComponentName
. For example:Canvas1FunctionName();
.
JSPaint
Renders the canvas, calling the JavaScript code defined on the event handler for OnPaint. You must pass it the context of a Canvas component (ComponentName_ctx).
Server-side Features
Properties, Methods and Events
- Documented in the RPCL Reference.
Help Resources
Tutorials
- HTML5 Canvas 2D Tutorial, by Al Mannarino.
- HTML5 Canvas 3D Tutorial, by Al Mannarino.
Video Tutorials
- HTML5 Builder support for Canvas, Storage & Audio/Video.
- 0:12 — Overview of CanvasPlanets.php.
Sample Applications
On the HTML5 folder inside the sample applications directory you will find two forms showcasing the Canvas:
- CanvasColorCircles.php. Interactive Canvas using a 2D context. It has settings to modify the content of the Canvas on the spot, and a button to generate a PNG file with the current content.
- CanvasPlanets.php. Adaption to the Canvas component of an official WebGL 3D example.
Documentation
- Standard API.
- Canvas on the Mozilla Developer Network’s wiki.
- Canvas on W3Schools.
- How to Draw with HTML5 Canvas, by Jamie Newman.
- Canvas on Opera’s Documentation.
- WebGL.
- Wiki.
- Documentation at the Mozilla Developer Network’s wiki.